Godot C# Tutorials⁚ A Comprehensive Guide
This guide provides a comprehensive exploration of Godot game development using the C# programming language. We will cover everything from setting up your environment to creating complex game logic‚ advanced techniques‚ and performance optimization. Join us on this journey to unlock the power of C# within the Godot engine.
Introduction to Godot and C#
Godot Engine is a powerful‚ open-source game engine renowned for its user-friendly interface and robust feature set. It empowers developers to create both 2D and 3D games for various platforms‚ including PC‚ mobile‚ and web. While Godot’s default scripting language is GDScript‚ it also offers support for C# through its Mono integration. This allows developers to leverage the familiarity and versatility of C# within the Godot environment.
C# is a modern‚ object-oriented programming language developed by Microsoft. It is known for its readability‚ strong typing‚ and extensive library support. C# is widely used in game development‚ particularly for larger-scale projects where its features and performance can be beneficial. Godot’s integration with Mono allows you to write C# scripts that interact directly with Godot’s engine and its rich set of nodes.
This tutorial series will delve into the world of Godot C# development‚ guiding you through the process of creating engaging games using this powerful combination. We will explore the fundamentals of C# scripting in Godot‚ cover essential concepts like working with nodes and implementing game logic‚ and dive into advanced techniques for optimizing your game’s performance.
Setting up Godot and C#
To embark on your Godot C# development journey‚ you need to ensure your environment is properly configured. First‚ download and install the latest stable version of Godot Engine from the official website. Once installed‚ you’ll need to enable Mono support. Navigate to the “Editor Settings” menu‚ then select “Mono” and enable the “Enabled” option. You might also need to install the .NET SDK‚ which provides the runtime environment for your C# code.
Next‚ you’ll need a suitable code editor or IDE to write your C# scripts. Visual Studio Code is a popular and free option‚ offering excellent support for C# development. Visual Studio‚ another Microsoft offering‚ provides a more comprehensive IDE experience. Within Godot‚ you can configure your external editor in the “Editor Settings” menu under “Mono” and “Editor.” This allows you to seamlessly switch between Godot and your chosen editor for code editing.
The setup process for Godot C# development might seem a bit involved‚ but it’s a one-time effort that sets the stage for a rewarding experience. Once your environment is ready‚ you can start crafting C# scripts and harness the power of this versatile language to create your own games within the Godot engine.
Creating a Simple C# Script
Let’s start with a basic C# script to get a feel for how things work in Godot. Create a new scene in Godot and add a “KinematicBody2D” node. This node represents a movable object in your game. Right-click on the “KinematicBody2D” node and select “Attach Script.” Choose C# as the language and name your script. A new C# file will be created in your project’s “Scripts” folder.
Inside your script‚ you’ll see the basic structure of a C# class. Add a method called “_Ready” that will be executed when the scene loads. Inside this method‚ we’ll add a simple line of code to print a message to the console. Use the following code snippet⁚
using Godot;
public class MyScript ⁚ KinematicBody2D
{
public override void _Ready
{
GD.Print("Hello from C#!");
}
}
Save your script and return to the Godot scene. Run the scene‚ and you’ll see the message “Hello from C#!” printed in the Godot console. This simple example demonstrates the basic process of writing and running a C# script within the Godot environment.
Working with Godot Nodes
Nodes are the fundamental building blocks of any Godot game. They represent objects‚ actions‚ and behaviors within your game world. To interact with nodes from your C# scripts‚ you can use Godot’s built-in API. This API provides methods and properties to access and manipulate the behavior of various nodes.
Let’s say you have a “Sprite” node in your scene and you want to change its texture. In your C# script‚ you can access the “Sprite” node using its name. The following code snippet demonstrates how to change the texture of a sprite node named “MySprite”⁚
using Godot;
public class MyScript ⁚ KinematicBody2D
{
public override void _Ready
{
var spriteNode = GetNode("MySprite");
spriteNode.Texture = GD.Load("res://my_new_texture.png");
}
}
This code retrieves the “MySprite” node using the “GetNode” method and then sets its “Texture” property to a new texture loaded from your project’s resources. This is just a basic example‚ but it demonstrates how you can interact with nodes to create dynamic game elements and behaviors within your C# scripts.
Implementing Game Logic
Game logic is the heart of your game‚ dictating how it behaves and responds to player actions. C# in Godot empowers you to implement complex game mechanics with its powerful features and robust libraries. You can use C# to control character movement‚ manage enemy AI‚ handle collisions‚ and implement scoring systems.
For instance‚ let’s consider a simple example of controlling a character’s movement with keyboard input. You can define a C# script attached to the player node that listens for key presses and updates the character’s position accordingly. Here’s a basic example⁚
using Godot;
public class PlayerMovement ⁚ KinematicBody2D
{
public float speed = 200f;
public override void _PhysicsProcess(float delta)
{
Vector2 velocity = Vector2.Zero;
if (Input.IsActionPressed("ui_right"))
{
velocity.x += 1;
}
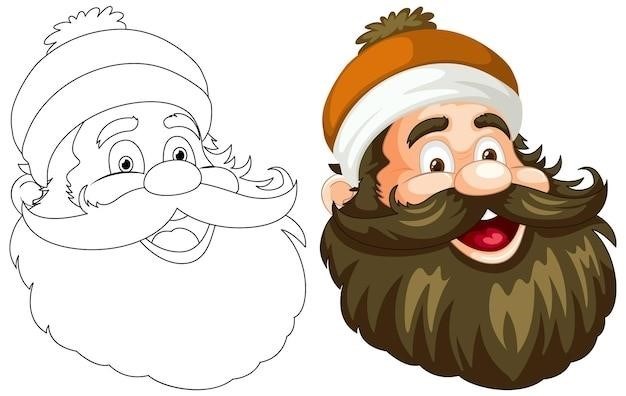
if (Input.IsActionPressed("ui_left"))
{
velocity.x -= 1;
}
if (Input.IsActionPressed("ui_down"))
{ velocity.y += 1;
}
if (Input.IsActionPressed("ui_up"))
{
velocity.y -= 1;
}
velocity = velocity.Normalized * speed;
MoveAndSlide(velocity);
}
}
This script uses the “Input.IsActionPressed” method to detect key presses and then updates the player’s velocity‚ which is then applied to the character using the “MoveAndSlide” function. By extending this logic‚ you can create intricate gameplay systems and behaviors that bring your game to life.
Advanced C# Techniques
As your Godot C# projects grow in complexity‚ you’ll need to leverage advanced techniques to enhance code organization‚ improve performance‚ and implement sophisticated features. C# offers powerful features like generics‚ delegates‚ events‚ and asynchronous programming that can significantly streamline your game development process.
Generics allow you to create reusable code structures that can work with different data types. For instance‚ you can create a generic “Inventory” class that manages items of any type‚ reducing code duplication and improving maintainability. Delegates and events enable you to decouple code components‚ allowing for more flexible and modular game design. You can use these to create custom events that trigger specific actions within your game‚ like a player reaching a certain point or an enemy being defeated.
Asynchronous programming‚ particularly with the “async” and “await” keywords‚ is essential for handling long-running operations like network calls or file loading without blocking the main thread. This ensures your game remains responsive and doesn’t freeze during these operations. By mastering these advanced C# techniques‚ you’ll be able to craft sophisticated game logic and mechanics with increased efficiency and elegance‚ unlocking the full potential of C# in your Godot development journey.
Performance Optimization
Optimizing your Godot C# games for smooth performance is crucial for delivering a satisfying player experience. While Godot’s engine provides a solid foundation‚ your C# code can significantly impact performance‚ especially as your game’s complexity grows. Here are key areas to focus on⁚
Profiling and Identifying Bottlenecks⁚ Use Godot’s built-in profiler to identify performance hotspots in your C# code. This tool helps pinpoint areas where excessive CPU or memory usage is occurring‚ allowing you to target your optimization efforts effectively.
Efficient Data Structures⁚ Choose appropriate data structures for your game logic. For example‚ use dictionaries for fast lookups‚ arrays for ordered collections‚ and linked lists for dynamic insertions and removals. Avoid unnecessary data copying and minimize object creation and destruction.
Code Optimization⁚ Optimize your C# code for efficiency. Minimize redundant calculations‚ use caching where appropriate‚ and consider using more performant algorithms. Avoid using unnecessary loops or recursive functions that can lead to performance degradation.
Memory Management⁚ Be mindful of memory usage. Utilize garbage collection effectively by releasing unused objects promptly. Consider using object pooling techniques to avoid frequent allocation and deallocation‚ which can impact performance. By strategically optimizing these aspects‚ you can ensure your Godot C# games run smoothly and deliver a polished player experience.
Debugging and Troubleshooting
Debugging and troubleshooting are essential skills for any game developer‚ and Godot provides a powerful set of tools to help you identify and resolve issues in your C# code. Understanding these tools and techniques can save you significant time and frustration during development.
Godot’s Debugger⁚ Godot’s built-in debugger allows you to step through your C# code line by line‚ inspect variables‚ and set breakpoints. This provides a powerful way to analyze the flow of your code and identify potential errors.
Console Output⁚ The Godot console provides a valuable way to output debugging information from your C# scripts. Use the `GD.Print` method to print messages‚ variables‚ or error details to the console‚ helping you track the execution of your code and identify problems.
Error Handling⁚ Implement robust error handling in your C# code. Catch exceptions gracefully and provide informative error messages. This helps you identify and resolve issues before they impact your game’s functionality.
Log Files⁚ Enable logging in Godot to record detailed information about your game’s execution. This can be helpful for tracking down subtle issues or performance bottlenecks. Analyze log files to gain insights into the behavior of your game and identify areas for improvement.
Community Resources and Support
The Godot community is a vibrant and welcoming space for game developers of all levels. It offers a wealth of resources and support to help you learn C# and master Godot game development. Here are some key resources you should explore⁚
Godot Forums⁚ The official Godot forums are a fantastic place to ask questions‚ share your projects‚ and engage with other developers. You’ll find a wealth of knowledge‚ helpful discussions‚ and experienced users willing to assist you.
Godot Discord⁚ The Godot Discord server is a bustling hub for real-time communication and collaboration. Join the conversation‚ ask questions‚ and connect with other developers in a friendly and supportive environment.
Godot Asset Library⁚ This library offers a vast collection of free and paid assets‚ including models‚ textures‚ scripts‚ and sound effects. Explore the library to find resources that can enhance your game development process.
YouTube Tutorials⁚ Many talented developers create YouTube tutorials specifically for Godot C# development. Search for tutorials on specific topics or techniques to expand your knowledge and learn from experienced creators.
GitHub Repositories⁚ GitHub is a valuable resource for finding open-source Godot C# projects and code examples. Explore repositories to learn from other developers’ work‚ discover new techniques‚ and contribute to the open-source community.
Embarking on a journey with Godot C# opens doors to a world of creative possibilities. You’ve gained a solid foundation in the fundamentals of setting up your development environment‚ crafting scripts‚ and working with Godot’s robust node system. Now‚ you’re equipped to tackle complex game logic‚ explore advanced C# techniques‚ and optimize your game’s performance for a seamless user experience. Remember‚ the key to success lies in continuous learning‚ experimentation‚ and embracing the collaborative spirit of the Godot community. Don’t be afraid to experiment‚ ask questions‚ and share your creations with the world. Godot and C# are powerful tools; use them to bring your game ideas to life and inspire others along the way.